How to Resize Specific Product Image in Magento 2 Using Command
In Magento 2, the product images are resized and stored in the cache after the product is saved. The storefront displays the images from the cache. If you’ve imported products or modified the images in the cache, you can use the Magento 2 image resize command to resize all images in Magento 2.
But what if you want to resize only specific product images in Magento 2?
The default image resize command in Magento 2 has no other parameters and does not support resizing only specific images. However, this can be accomplished by creating a CLI command in Magento 2.
I created a custom console command in Magento 2 to resize specific product images. In this post, you’ll find the complete steps + code to create the command.
Let’s learn to resize specific product image in Magento 2 using command.
Create a Custom Console Command to Resize Product Images by ID in Magento 2
To create a custom console command in Magento 2, we’ll need to create a module and define it.
Follow these steps to do that:
Create a module.xml file at app/code/Vendor/Extension/etc/ directory and add the following code:
1 2 3 4 5 6 7 8 |
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework/Module/etc/module.xsd"> <module name="Meetanshi_SingleProductImageResize" setup_version="1.0.0"> <sequence> <module name="Magento_Catalog"/> </sequence> </module> </config> |
Now, create di.xml file in the same directory with the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd"> <type name="Magento\Framework\Console\CommandListInterface"> <arguments> <argument name="commands" xsi:type="array"> <item name="imagesResizeCommand" xsi:type="object"> Meetanshi\SingleProductImageResize\Console\Command\ImagesResizeCommand </item> </argument> </arguments> </type> <type name="Meetanshi\SingleProductImageResize\Console\Command\ImagesResizeCommand"> <arguments> <argument name="imageResizeScheduler" xsi:type="object"> Magento\MediaStorage\Service\ImageResizeScheduler\Proxy </argument> </arguments> </type> </config> |
Register the module by adding registration.php file in the app/code/Vendor/Extension/ directory with the following code:
ImageResizeCommand.php at the app/code/Vendor/Extension/Console/Command/ directory and add the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 |
<?php declare(strict_types=1); namespace Meetanshi\SingleProductImageResize\Console\Command; use Magento\Framework\App\Area; use Magento\Framework\App\State; use Magento\Framework\Console\Cli; use Magento\MediaStorage\Service\ImageResize; use Magento\MediaStorage\Service\ImageResizeScheduler; use Symfony\Component\Console\Helper\ProgressBar; use Symfony\Component\Console\Helper\ProgressBarFactory; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; use Symfony\Component\Console\Input\InputOption; use Symfony\Component\Console\Command\Command; use Symfony\Component\Console\Input\InputArgument; use Magento\Framework\DB\Query\Generator; use Magento\Framework\DB\Select; use Magento\Framework\App\ResourceConnection; use Magento\Catalog\Model\ResourceModel\Product\Gallery; use Magento\Catalog\Model\ResourceModel\Product\Image as ProductImage; /** * Resizes product images according to theme view definitions. */ class ImagesResizeCommand extends Command { /** * Asynchronous image resize mode */ const ASYNC_RESIZE = 'async'; /** * @var ImageResizeScheduler */ private $imageResizeScheduler; /** * @var ImageResize */ private $imageResize; /** * @var State */ private $appState; /** * @var ProgressBarFactory */ private $progressBarFactory; /** * @var ProductImage */ private $productImage; /** * @var ResourceConnection */ private $resourceConnection; public function __construct( Generator $generator, ImageResizeScheduler $imageResizeScheduler, State $appState, ImageResize $imageResize, ProgressBarFactory $progressBarFactory, ProductImage $productImage, ResourceConnection $resourceConnection ) { parent::__construct(); $this->batchQueryGenerator = $generator; $this->imageResizeScheduler = $imageResizeScheduler; $this->appState = $appState; $this->imageResize = $imageResize; $this->progressBarFactory = $progressBarFactory; $this->productImage = $productImage; $this->resourceConnection = $resourceConnection; } /** * @inheritdoc */ protected function configure() { $this->setName('image:product:resize') ->setDescription('Single Product Image Resized') ->setDefinition($this->getOptionsList()); $this->addOption( 'product_id', null, InputOption::VALUE_REQUIRED, 'Add Product ID' ); } /** * Image resize command options list * @return array */ private function getOptionsList() { return [ new InputOption( self::ASYNC_RESIZE, 'a', InputOption::VALUE_NONE, 'Resize image in asynchronous mode' ), ]; } /** * @inheritdoc * @param InputInterface $input * @param OutputInterface $output */ protected function execute(InputInterface $input, OutputInterface $output) { $product_id = $input->getOption('product_id'); $result = $this->executeAsync($output, $product_id); return $result; } /** * Schedule asynchronous image resizing * @param OutputInterface $output * @param int $product_id * @return int */ private function executeAsync(OutputInterface $output, $product_id) { try{ $errors = []; $this->appState->setAreaCode(Area::AREA_GLOBAL); /** @var ProgressBar $progress */ $progress = $this->progressBarFactory->create( [ 'output' => $output, 'max' => $this->getCountUsedProductImages($product_id) ] ); $progress->setFormat( "%current%/%max% [%bar%] %percent:3s%% %elapsed% %memory:6s% \t| <info>%message%</info>" ); if ($output->getVerbosity() !== OutputInterface::VERBOSITY_NORMAL){ $progress->setOverwrite(false); } $productImages = $this->getUsedProductImages($product_id); foreach ($productImages as $image) { $result = $this->imageResizeScheduler->schedule($image['filepath']); if (!$result){ $errors[$image['filepath']] = 'Error image scheduling: ' . $image['filepath']; } $progress->setMessage($image['filepath']); $progress->advance(); } } catch (\Exception $e){ $output->writeln("<error>{$e->getMessage()}</error>"); return Cli::RETURN_FAILURE; } $output->write(PHP_EOL); if (count($errors)){ $output->writeln("<info>Product images resized with errors:</info>"); foreach ($errors as $error){ $output->writeln("<error>{$error}</error>"); } } else{ $output->writeln("<info>Product images scheduled successfully</info>"); } return Cli::RETURN_SUCCESS; } /** * Get used product images. * @param int $product_id * @return \Generator */ private function getUsedProductImages($product_id) { $batchSelectIterator = $this->batchQueryGenerator->generate( 'value_id', $this->getUsedImagesSelect($product_id), 100, \Magento\Framework\DB\Query\BatchIteratorInterface::NON_UNIQUE_FIELD_ITERATOR ); foreach ($batchSelectIterator as $select){ foreach ($this->resourceConnection->getConnection()->fetchAll($select) as $key => $value){ yield $key => $value; } } } /** * Return select to fetch all used product images. * @param int $product_id * @return Select */ private function getUsedImagesSelect($product_id) { $query = 'images.disabled = 0 AND image_value.disabled = 0 AND image_value.entity_id = '.$product_id; return $this->resourceConnection->getConnection()->select()->distinct() ->from( ['images' => $this->resourceConnection->getTableName(Gallery::GALLERY_TABLE)], 'value as filepath' )->joinInner( ['image_value' => $this->resourceConnection->getTableName(Gallery::GALLERY_VALUE_TABLE)], 'images.value_id = image_value.value_id', [] )->where($query); } /** * Get the number of unique and used images of products. * @param int $product_id * @return int */ private function getCountUsedProductImages($product_id) { $select = $this->getUsedImagesSelect($product_id) ->reset('columns') ->reset('distinct') ->columns( new \Zend_Db_Expr('count(distinct value)') ); return (int) $this->resourceConnection->getConnection()->fetchOne($select); } } |
That’s it. You can now use this custom module to resize specific product images in Magento 2 using command.
How to Resize Specific Product Images in Magento 2 Using Command
You can use the following command to resize specific product images in Magento 2:
php bin/magento meet:product:resize --product_id=33
You can pass any product ID in the command above and resize a specific image in Magento 2.
& that’s it!
Loved this post? Please share it with your friends on social media & spread the knowledge.
In case you’ve any doubts or queries, feel free to comment.
Thank You!
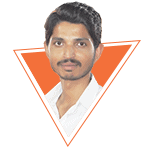
Jignesh Parmar
An expert in his field, Jignesh is the team leader at Meetanshi and a certified Magento developer. His passion for Magento has inspired others in the team too. Apart from work, he is a cricket lover.
2 Comments
Hi ,
I have used your module but it is not replaced the existing image of the product with new one.
Can you please help in in it.
Thanks
Hey Aakash,
By the information given in the blog
you can resize the images.
You cannot change/replace the images.